Build Classified Ads Project With ReactJS: A Step-by-Step Guide
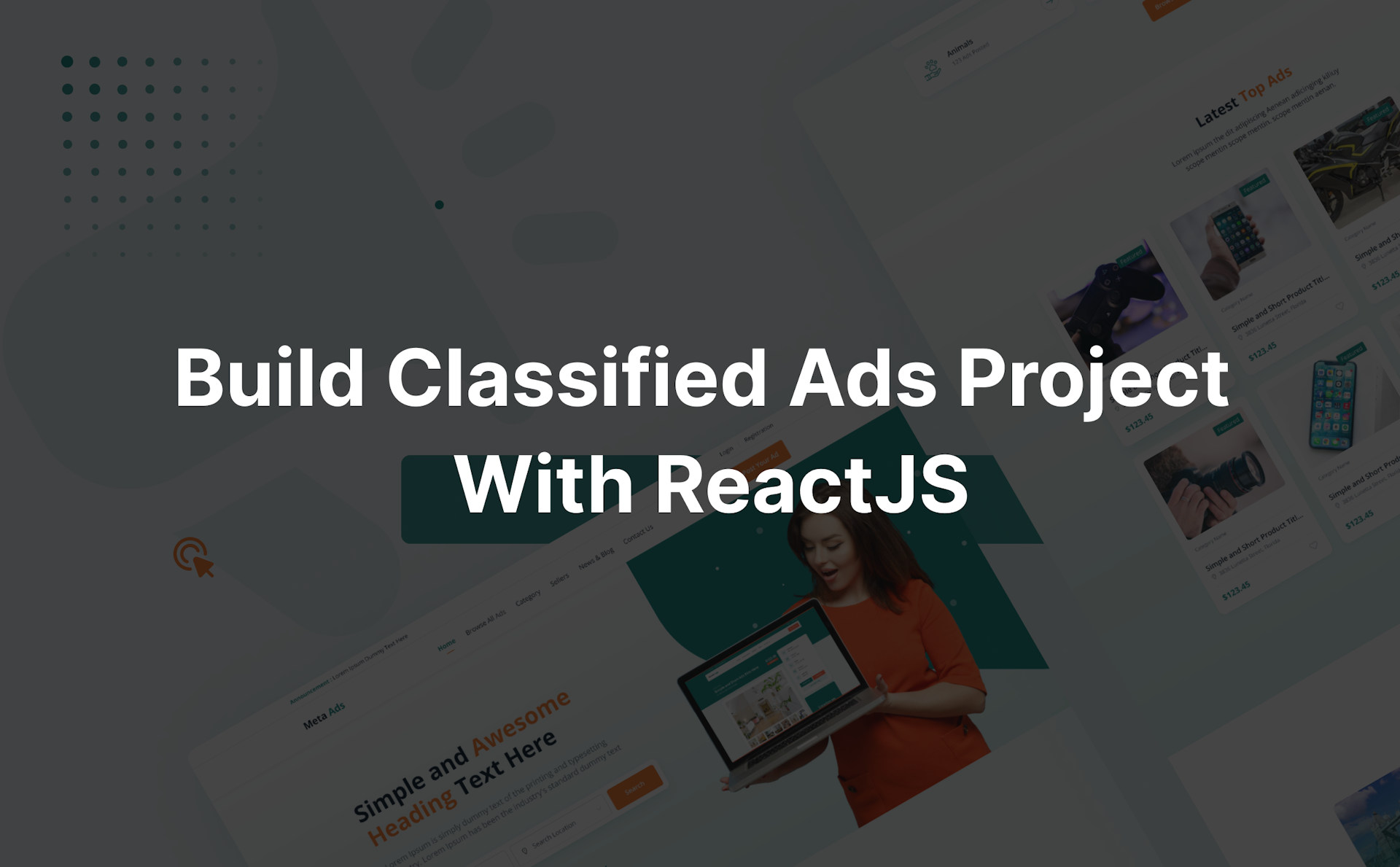
You're a web developer looking to build a classified ads site, but want it to be fast, scalable, and use modern tools. ReactJS is a great fit! In this guide, I'll walk you through building a classifieds project from start to finish using ReactJS and NextJS. In 100 words or less, you'll learn how to set up a React app, build reusable components, fetch data from an API, implement routing and pagination, optimize performance, and deploy to production. With some coding experience under your belt, you'll be able to follow along and have your own classifieds site up in no time. The end result will be a lightening fast, SEO-friendly, and scalable classifieds web app you can be proud of and use as a portfolio piece. Let's dive in!
Overview of the Classified Ads Project
Building a classified ads project with ReactJS allows you to create a fast, scalable web app for posting and browsing local listings. This guide will walk you through the major steps to build the project using React, Next.js, and Headless CMS 👇
Prepare Documents
Prepare documents with all of your requirements. If you are planning a complex classified ads project and launching it globally, you should also have an MVP plan. So, preparing documents is important, and make sure you have them. It will help you make faster progress to initiate the project.
Design the Data Model
The first step is determining what data you need to store for each listing. At a minimum, you'll want a title, description, price, photos, and contact info. You may also want location data, categories, and more.
Set Up the Backend
Next, build an API to manage the data using Next.js and MongoDB. Create endpoints to handle CRUD operations on listings, uploading images, and searching. Use environment variables to keep your database URL and other secrets private.
Build the Frontend
Now you can start building the frontend React app! Create pages to view listings, create a new listing, edit a listing, and more. Use React Bootstrap or Material UI to style the pages. Allow users to filter and search listings.
Add Features
Some other useful features for a classifieds site include:
âś… User accounts so people can save listings and manage their posts âś… Email notifications when new relevant listings are posted âś… Location-based search to find listings close by âś… Ratings and reviews to build trust in the community âś… Chat or messaging to allow buyers and sellers to communicate
Key Features to Include in a Classified Ads React App
Download the Ready Classified Ads project. It's fully lightning fast, secure, and scalable. Built with Headless CMS Strapi, React.js, Next.js, and Postgres.
User profiles
Allow users to create profiles to post ads, favorite ads, message sellers, leave reviews, and more. User profiles add a social element to your classifieds app and help build community.
Ad categories and listings
You'll want to include categories like vehicles, real estate, jobs, and general for sale. Within each category, allow users to post listings with photos, descriptions, locations, and contact info. Consider offering paid "featured" ad spots and allow users to bump their listings to the top.
Search and filters
A robust search feature is essential for a classifieds app. Allow users to filter by category, location, price, keywords, and other options. Advanced filters, like searching within a specific city radius or price range, make it easy for buyers to find what they need.
Messaging
Include a messaging system so buyers and sellers can communicate privately. This is an important feature for facilitating transactions and building trust in your community. Consider offering notifications to alert users of new messages.
Reviews and ratings
Reviews and ratings help establish reputations and build trust. Allow users to leave reviews for each other after a transaction. Reviews should be tied to a user's profile and also appear on their individual ad listings.
Saved ads and favorites
Many classified app users regularly check the listings for items they're interested in. A saved ads feature allows them to bookmark listings they like so they can check back on the status or be notified if the price changes. Saved ads encourage repeat usage of your app.
By including these key features in your React classified ads project, you'll have an app that helps bring buyers and sellers together, builds community trust, and keeps people coming back. The specific implementations are up to you, but focusing on profiles, listings, search, messaging, reviews and saved ads will result in an app that serves user needs well. Good luck building your project!
Choosing the Right Reactjs Template and Theme


Simplicity is Key
When choosing a React template for your classified ads project, opt for simplicity. Look for a minimal, lightweight template without too many pre-built components or styling. You want a template that provides a good starter structure and file organization, but allows you to build out the UI and functionality for your specific needs. Some great options are Create React App, React Starter Kit, and React Redux Starter Kit. These are minimal, flexible templates perfect for building your app from scratch.
Mobile-First Matters
Since many users will be accessing your classified ads site from their mobile devices, choose a template with a mobile-first approach. This means the template is designed for optimal viewing on smaller screens, then scales up nicely for larger desktop screens. The styling and components will work seamlessly on any device. Some templates advertise “responsive” design, but mobile-first is really what you want.
Styled With Your Needs in Mind
Some templates come with pre-built styling and UI components that you have to work around. It’s better to choose a template with minimal styling so you can craft the look and feel to match your app’s needs.Think about the overall style you want—minimal and modern or more traditional. Consider your brand’s colors and typography. The template should make it easy to implement your own styling from scratch. You want full control and flexibility over the UI.
Scalability and Performance
Since your classified ads app needs to handle many listings and users at scale, choose a template optimized for performance and scalability. Look for templates built with React Hooks, React Context API, and React Suspense for data fetching. These features will ensure your app stays fast and responsive under heavy load. Your template should also support server-side rendering with Next.js for SEO and initial page load performance.
Choose your React template wisely and you’ll have a solid foundation to build an scalable classified ads app. Keep it simple, go mobile-first, and retain full control over styling and UI. With the right template, you can focus on crafting a great user experience and building out the functionality to match your vision.
Optimizing Performance in a Classifieds React App
To ensure fast loading times and a smooth experience for users, performance optimization is key for any React app, especially one at scale like a classifieds site.
Use Server-Side Rendering
With Next.js, you get server-side rendering (SSR) by default. This means that pages are pre-rendered on the server, then sent as HTML to the client. This allows for faster loading and better SEO since crawlers have access to rendered HTML content.
Lazy Load Components
In a classifieds app, you'll likely have many components that aren't needed right away on page load, such as listing details or seller profiles. Use React's lazy() and Suspense API to lazily load these components only when needed. This can significantly improve your app's performance and loading times.
Optimize Image Loading
Images are often the biggest culprit for performance issues in React apps. Be sure to:
Load only optimized image sizes needed for the viewport
Use modern image formats like WebP and AVIF
Apply placeholders until images finish loading
Consider using a CDN to serve images
By following these optimizations, you'll build a fast, scalable classifieds app in React that provides a great experience for your users. Performance should always remain a top priority, so continue profiling and improving as your app grows.
Deploying the Classified Ads App
Choose a Hosting Platform
When it comes to deploying a React app, you have a few good options. Two of the most popular are Netlify and Vercel (formerly ZEIT Now). Both offer generous free plans and make deploying React apps incredibly simple. For this project, we'll use Vercel.
Connect to Vercel
Head to Vercel.com and connect your project's GitHub repo. This will automatically detect that you have a React app and configure the build settings for you.
Set Environment Variables
If your app uses any sensitive keys or environment variables, you'll want to configure those in Vercel now. Go to the "Environment Variables" section in your project settings and add any keys your app needs.
Review and Deploy
Vercel will now build a preview deployment of your app that you can view and test. Check that everything looks good, then click "Deploy" to make your app live! Your app will be live at a URL like your-app-name.vercel.app.
Custom Domain (Optional)
If you have your own custom domain, you can point its DNS records to Vercel to use that domain instead. Go to the "Domains" section, add your domain, and Vercel will provide the DNS records you need to configure. Once the domain is verified in Vercel, your app will be live at that custom domain.
Continuous Deployment
One of the best parts of Vercel is that it enables continuous deployment from GitHub. This means that any time you push an update to your repo, Vercel will automatically rebuild and redeploy your app. No more manual deployments needed!
Between the generous free plan, simple setup, and continuous deployment, Vercel makes deploying and updating your React apps an absolute breeze. Your classified ads app will be live and updating automatically in no time. Let me know if you have any other questions!